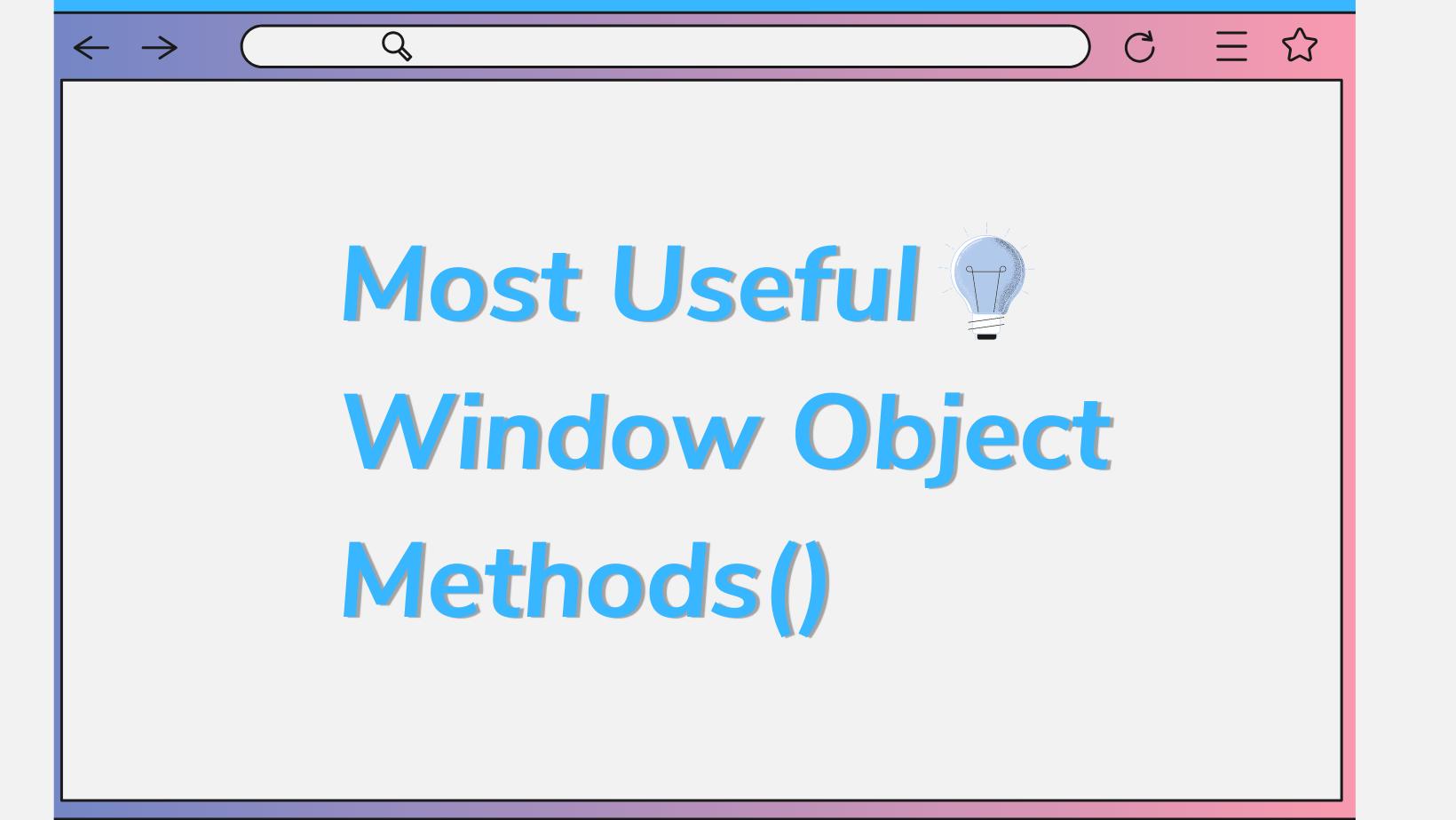
One of the key elements of JavaScript DOM is the global Window Object. It provides a wide range of methods and objects that can be incredibly useful for web developers. In this article, we will explore some of the most frequently used features of the Window Object that can greatly enhance your coding experience.
1. setTimeout()
The first method we will discuss is setTimeout()
. This method allows you to execute a function after a specified delay. It takes two arguments: a callback function and a time in milliseconds.
Imagine if you have to display a success or error modal after submitting a Form after a certain period or if you are just working on a Project Locally and want to imitate a fetch request from an API. Well, the setTimeout()
method is the way to go.
In the given example, after a page is loaded, the customTheme() function is called after a 5-second delay. It displays a confirmation modal and prompts the user to input a colour to change the website's background. If a valid colour is entered, it applies the colour, hides a toggle button, and updates the heading text.
2. setInterval()
Often, we need to repeatedly execute a function at specific intervals. For such scenarios, `setInterval()` comes to our rescue.
This method takes a function and the interval at which the function should be executed.
But what if you want to stop the function after certain intervals?
Well, there's also a method for just that. The clearInterval()
takes the timer or basically a variable in which the value of setInterval()
is stored and stops/clears the function inside setInterval()
.
In the above example, the startTimer()
function initializes a timer. It sets the initial value of the timer element to 0 and starts a setInterval()
function. This setInterval()
function updates the timer element every second by calling the getTimerTime()
function.
NOTE: However, it is important to note that setInterval()
may not be precise due to the execution time of the function it calls.
To achieve more precise delays at regular intervals, it is recommended to use a nested setTimeout()
function instead. For a detailed explanation of the differences and use cases, refer to this article.
3. scrollTo()
One of the most Used window methods. No website today is complete without a Back to Top button. I mean who doesn't love one such button at the footer of a website which when clicked saves you the hazard of using the Scrollbar to get back to the top of the Page? Also, it makes navigating through different sections of your website easy and neat which also increases the user experience. With `scrollTo()`, you can implement this functionality with a single line. The method takes two arguments, the X and Y (horizontal and vertical) coordinates of where you want to scroll to on the page. the value cannot be negative.
The below example shows a button which takes the user to the top of the Page when clicked.
Use this with the CSS below for enabling smooth scrolling and you are good to go.
4. Window.open() / Window.close()
The open()
method of the Window Object is used to open a new browser window or tab.
It takes the following parameters:-
- url (optional): The URL of the page to be opened. If not specified, an empty window will be opened.
- name (optional): The name of the window or tab. It can be used as a target for links and form submissions. If not specified, a new window will be opened with a default name.
- features (optional): A string of window features such as size, position, and toolbar visibility. These features are comma-separated and can be combined.
In the above example, the openNewWindow()
function is called when a button is clicked. It uses the open()
method to open a new browser window or tab with the URL https://www.example.com The "_blank
" parameter specifies that the page should be opened in a new tab, and the "width=800,height=600"
parameter sets the size of the new window. You can also pass an argument as popup
to it and it will open the new window in a popup-like fashion just like how Google OAuth does it.
Similar to the open()
method window.close()
method of the Window Object is used to close the current browser window or tab. I know we are talking about methods, but the next one is an object
5. Location Object
The location object provides information about the current URL and allows you to navigate to different URLs. It contains properties and methods that can be used to manipulate the browser's location.
Properties:
- href: Returns the complete URL of the current page.
- host: Returns the hostname and port number of the current page.
- protocol: Returns the protocol
(http:// or https://)
of the current page. Can be helpful when managing development and production environments in your code.
- pathname: Returns the path or filename of the current page, and can be used to track and perform actions depending on the current pathname of your website.
- search: Returns the query string of the current page. Very important if your code depends on the query parameters of the URL, one common use case is fetching paginated Data from your server depending on which page number you are at.
- hash: Returns the anchor part of the URL.
Methods:
- assign(url): Loads a new document by setting the href property to the specified URL. helpful in navigating through different pages programmatically.
- reload(): Reloads the current document, helpful in manually submitting a form or fetching fresh data from the server.
- replace(url): Replaces the current document with a new one by setting the href property to the specified URL
6. Local Storage
This last one is also an Object with very useful methods. How many times have you got your UI changes destroyed (removed) after reloading the Page? All your data with your hard work to make the app work, is Gone. Therefore if you want to persist with the changes you made to your website even after you reload the website, you'll need this object method. Like all Objects _localStorage_ stores key-value pairs and almost all object methods work on it like any other plain Object. It mainly uses two methods which are localStorage.setItem()
and localStorage.setItem()
to save and retrieve a value from the localStorage Object. The Earlier takes key and value as arguments while the latter only takes the key. The keys and values stored in the localStorage object can only be strings. So if you want to store objects within the localStorage you can use the JSON.stringify()
method below :
The above code sets a value in the local storage by assigning a stringified JSON object to the "form-data" key. To retrieve the data, the JSON.parse() method is used to parse the stored value. In this case, the "name" property of the object is displayed in the console.
There's also a localStorage.removeItem(key)
which removes a specific item from the storage by its key. Additionally, you can also clear the localStorage by calling localStorage.clear()
to remove all the keys in our localStorage
Some common use cases of localStorage include saving formState of huge forms between page reloads, persisting the theme preferences of your website, and sometimes saving an Authorization Token sent from an API to the localStorage and retrieving it later to authenticate the user. I generally don't recommend this and prefer httpOnly cookies over localStorage to save my sensitive information
Also, remember that local storage only allows you 2MB of maximum storage space, so if your data requirements are larger than that, then you should look for other browser-based storage solutions like indexed DB and the Cache API
The Window Object offers a plethora of features to enhance JavaScript DOM programming. In this article, we only scratched the surface. By leveraging these features, you can create more interactive and user-friendly web applications. Stay tuned for future articles where we dive deeper into the magical world of the Window Object.
Until Next Time,
Happy Debugging Coding👋