How I write code after 3 years in the Software Industry
Ok I've been here for some time and I have opinions
Or as the cool kids say nowadays
Started from the bottom, now we're here
First, let's get one thing clear, this post is not for overwhelming or disheartening beginner software developers. In fact, as a genius Engineer once said:
you should write bad code to write good code
- Some random soy JS dev (probably me)
All of us have been there. I have written so much bad code that compared to it, running useEffect recursively doesn't sound that big of a deal๐.
This post is for newer devs to start thinking about from a different point of view while developing software, and maybe change their approach and mindset to think about scale, outages, traffic, performance, testing and other common yet important concepts.
So let's look at some of the common developer dilemmas and strategies to solve them at a high level and then let's dig into some coding examples to compare the code
The best stack is the one you're experienced with
If you've seen the framework wars on Twitter that have been going off for decades, you know that (insert your favourite stack here) is the GOAT.
Especially in the JS ecosystem, every day is a day when a new Blazingly Fast, Game-Changing JS Framework, Auth library, JS Runtime or Build Tool is born.
I've also been part of this hype train when I started off. I've tried and played around with almost every new Framework, Library, and even databases and languages for weeks and it is so fun for me from the perspective of learning and understanding different concepts and ways of solving problems. I genuinely enjoyed the time I spent on this.
So if you're also passionate about this, sure give it a try. But if your goal is to get a Job, Learn Which Technology is in demand in your area, learn it, follow its coding practices, use its libraries and tech stacks and GET PAID.
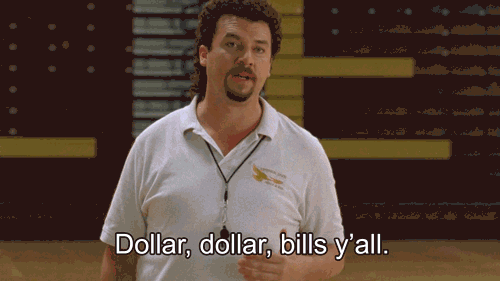
On the contrary, You must have watched or heard about the discussion going on about the opinions of Peter Levels on the Lex Fridman Podcast
My 2 cents on this is in the heading of this section and this๐
Don't take advice from random strangers on the internet
who shove their biased opinions masked as advice and claim that this new cool thing is THE FUTURE of Development.
Meanwhile, 70% of websites still run on PHP and the PHP devs are like
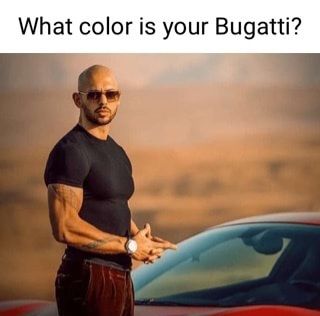
If you are an Indie hacker or just a beginner dev trying to get better and faster at coding. Stop switching technologies and just pick one that you enjoy the most right now
You can implement similar design patterns and best practices in any programming language and framework.
The tough choice is to weigh the pros and cons that your existing stack is right to solve this specific problem and investing the time and effort to learn this new shiny thing is worth it and eventually gonna pay it off in the long run.
Use Typescript
Of course, If you are a JS dev. If not what the hell are you doing on my Blog?
JS was originally made as a dynamic scripting language for the web and I know that with NodeJS we have a great ecosystem for server-side development with JS but the idea of JS running on the server didn't really sit well with me.
Static Type Checking is essential in any server-side language for me.
With TS, I can write better, safer code and don't have the urge to blow my head off any time I see cannot read properties of undefined (reading 'yourmom')
and TS prevents this by converting a dynamic language into a Static Type-Safe one.
It also gives you nice IntelliSense in your IDE while accessing object properties and enforces strict yet customizable rules like null checking, params and return types while writing code so you can catch bugs right in the editor instead of runtime. Almost every new library, framework and now even runtimes like deno and bun just come with TS support out of the box.
Nowadays I write TS even if I'm building a SPA client app with any UI framework. Modern Full-Stack frameworks like NextJS, Remix, Astro etc. along with ORMs like Prisma, Drizzle and Zod for validation make it even more frictionless to write TS on both client and server and ensure full type safety right from the database all the way to your UI giving a seamless DX along the way.
Now it's not all Guns and Roses or whatever the saying is.
If you're using NodeJS, You have to run an additional compile step that converts your TS files back to JS before you can use Node to serve your application and the compile time, well let's say it can be pretty slow at times and add a bit of complexity to your app but modern toolings like esbuild and TS supported runtimes like deno, Cloudflare, bun etc. solves this.
Oh and with TS, you can just
Skip non-essential Shit like Writing Tests
Yup, I said it, come at me Tech Twitter ๐๐
If you're too deep into JS and not ready to let it go, you can progressively adapt TS just as Microsoft intended for it to be used. You can try it on the server first and then move on to the client with the help of the full-stack framework mentioned above. Anyhow I highly recommend giving it a try before hating on it.
Even if this didn't convert you and all of this sounds like extra work, then at least check out JSDoc along with ESLint which will get you almost 70% of the way TS could.
Don't reinvent the wheel
You must have heard this before, but building something from scratch is a waste of time and effort, Your job as a Programmer is to solve problems faster and efficiently and it doesn't make sense to code every feature from scratch to achieve a common functionality unless if you're a FAANG Engineer in which case, Why are you doing here? You must have 10 tickets to raise and a dozen emails to send to your seniors before getting the approval to change the CTA button Color from black to White.
Expect them, it is always good to rely on tried and tested production-ready solutions instead of re-inventing the wheel.
I'd like to quote Myself that one genius engineer again.
Perfectionists don't make money, they argue on X about how PHP still sucks and how your app with 0 active users should be able to handle 1 million concurrent request per second.
Take Marc Lou for example, an Indie maker who shipped 25+ products in 3 years, made over a million dollars and uses doesn't use TS, doesn't test his code, has no git branching, ships directly to production and hasn't changed his boring Next, Tailwind, MongoDB and Vercel stack in 3 years.
Now if you're in a company, Don't be like Marc, test your code and Don't push to Prod.
The point I'm trying to make is You need to work with tools and languages you and your team are familiar with to ship and iterate faster. Even if the tools sound boring and your mind wants to jump to the next new hype on tech Twitter.
Remember,
It's doing the boring things over and over that helps you succeed.
Either use open-source tools as much as you can if your company allows it or use existing systems, processes and frameworks that your team has developed over the years.
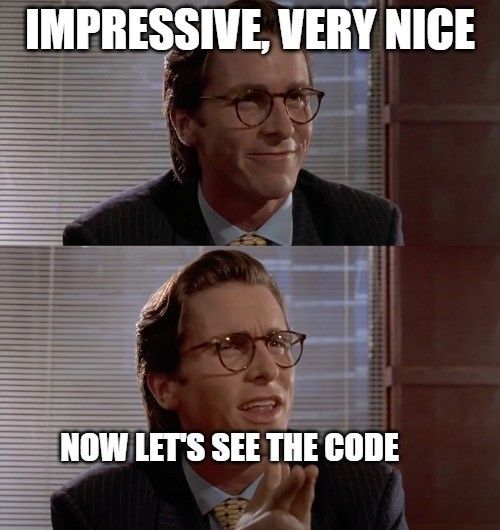
Let's compare the differences in the code choices between a Junior and a Senior Dev
The below code snippets are written in JS/TS but the concepts are the same and can be converted in any programming language.
1. Caching:
Server-side caching is crucial for optimizing performance, especially for high-traffic endpoints. Proper caching strategies can significantly reduce server load and improve response times. Junior devs often overlook caching, leading to performance bottlenecks.
While Senior devs use some sort of memory store, Redis in our case first check if we have the in-demand data in memory or not, If there's no data only then we hit our primary db.
Bonus: You can also use redis to rate limit requests going to your server so that attackers cannot spam your server with consecutive requests. It integrates very well with the express-ratelimit
and many other nodejs packages.
Because frankly I don't get why someone would still use express in 2024 when you have so many good options today but to each their own.
2. Querying Data:
Querying data effectively is very important for decreasing API response time specially when handling large datasets. Junior developers often fetch all data at once, causing performance issues. They also don't utilise indexes to speed up their queries.
Senior developers implement pagination along with indexes while querying large datasets to fetch a specific range of records instead of all of them and index certain columns for faster retrieval of indexed records. This is helpful in optimizing data fetching and reducing server load.
3. Data Mutations:
Handling Data Mutations on the server is a crucial part that you have to think about while writing code that scales. Whenever you update multiple tables in your database, you don't want to be stuck in a state where some records update while others fail.
Transactions are a way to perform multiple mutation actions one by one which provides an effective way to instantly roll back the database state in case any of the actions fail. This ensures data integrity during multiple database operations.
Junior developers often skip transactions, risking data inconsistency.
Senior developers use transactions to handle complex data mutations safely ensuring data consistency and integrity.
4. Server logs and Error Monitoring:
Effective logging and error monitoring are essential for maintaining application health and diagnosing issues.
Junior developers might implement basic logging without structured formats or centralized monitoring. You don't want to be in a situation where a user has to inform you that your app stopped working.
This is what logging in a junior dev's codebase looks like. Now if anyone on the team wants to check logs, they have to SSH into the VPS, run pm2 logs or whatever else you are using and see the logs manually and hope to god that they resolve the issue fast before other complaints start flying in.
Senior developers use advanced tools like for structured logging, centralized monitoring, and alerting through Slack, discord or email channels.
You can use any of the popular logging and monitoring services like Datadog, logstash, posthog, sentry etc.
We're using Loki Grafana in the below example.
We only need to set a logging middleware in our web app and through the Grafana dashboard, we can customise where we want to receive our alerts.
5. Database migrations, Replicas and Backups:
While Juniors always leave everything up to chance. Senior developers always manage database migrations effectively, have replicas in place for the production db and backup old data at regular intervals to multiple data centres at different locations. To learn more about these database-related topics in detail, check out my latest post on this topic.
6. Testing:
This may be a counter-intuitive take but I don't do unit or integration testing for my products, most of the companies I've worked with either had a dedicated testing team or only did End-to-end tests so I don't have much of an opinion on this. Maybe will write a post on how to do e2e testing in the future.
For now, my take is if writing tests doesn't slow you down, only then you should do it.
Otherwise, good PR reviews, a good setup of development and staging environments, and Unopiniated coding practices are good enough reasons for you not to write tests.
This paired with the above points we discussed in this post will increase your code quality, If you still need tests, You have a skill issue buddy.
Well, Enough Opinion for one post.
Until Next Time,
Happy Debugging Coding๐